1.IP和InetAddress#
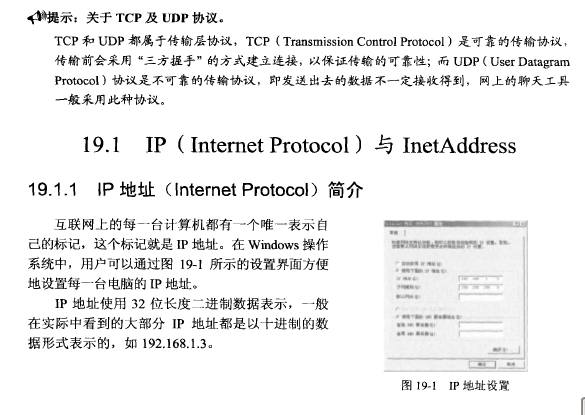
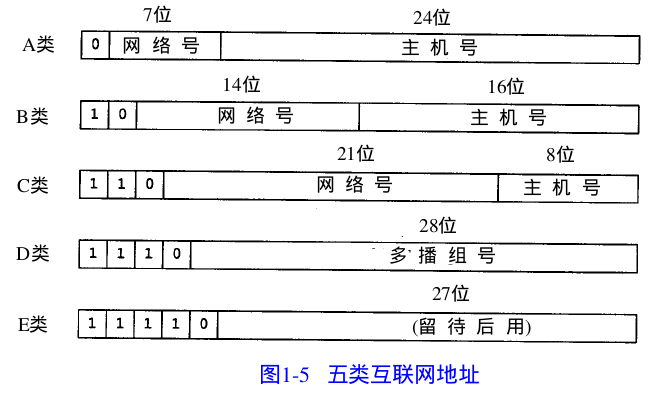
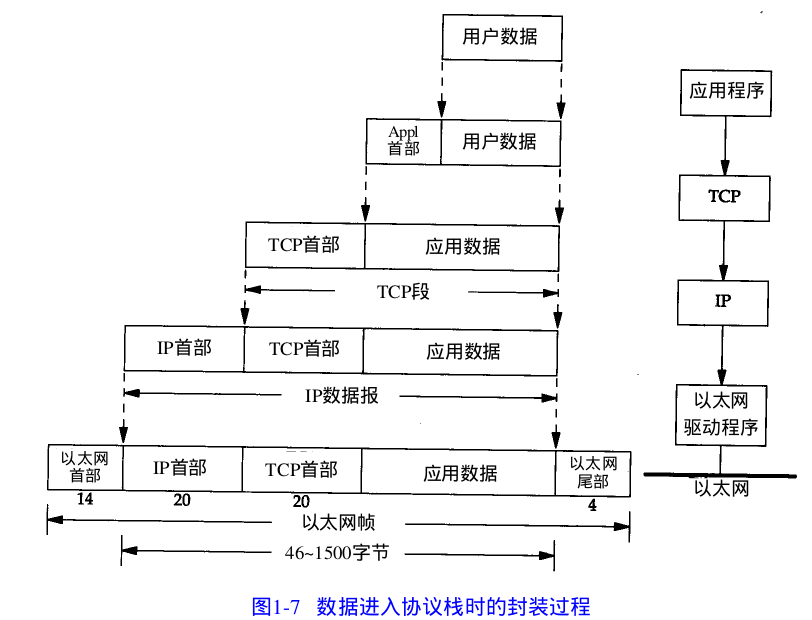
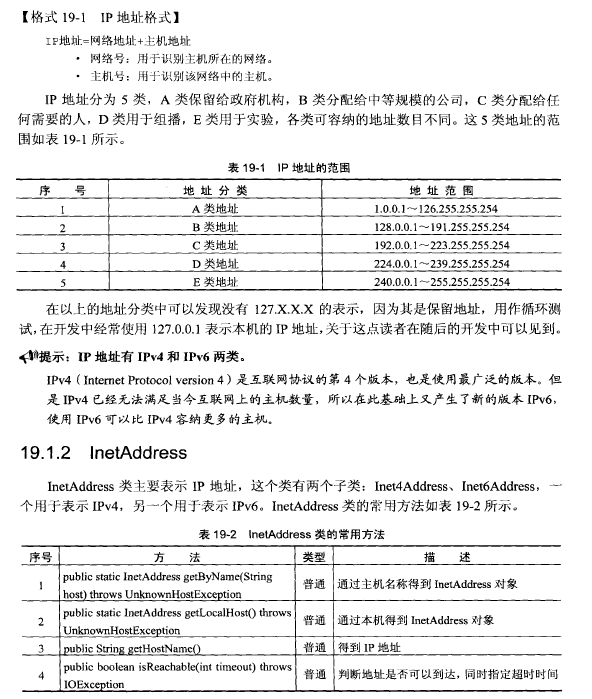
1 | import java.net.InetAddress; |
2.URLConnection,URLEncoder和URLDecoder#
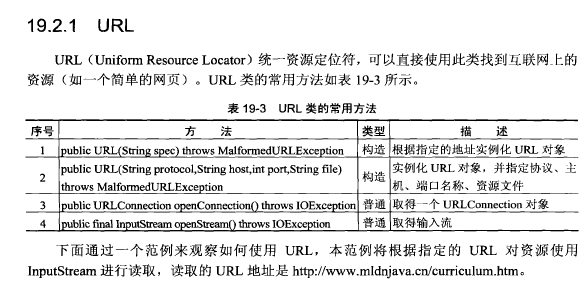
1.使用URL读取内容#
1 | import java.awt.im.InputContext; |
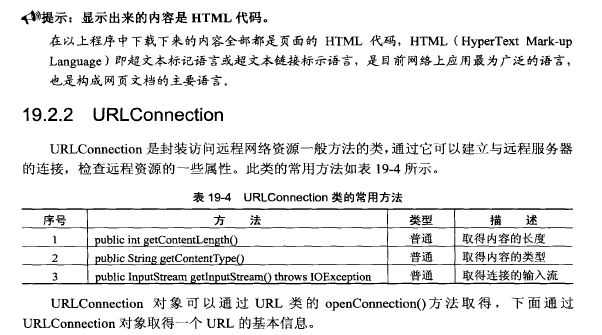
2.取得URL的基本信息#
1 | import java.net.URL; |
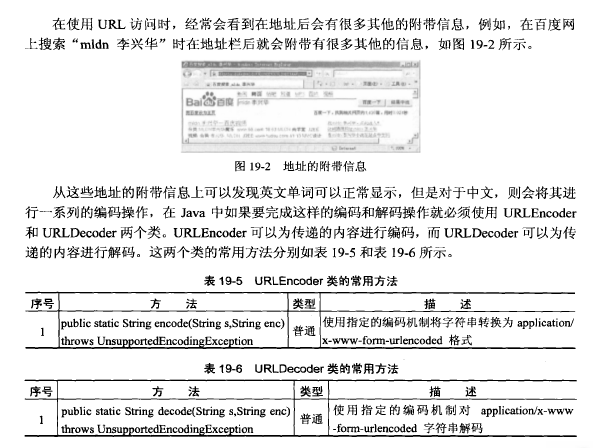
1 | import java.net.URLDecoder; |
输出
1 | 编码之后的内容:%E5%BC%A0%E4%B8%89 |
3.TCP#
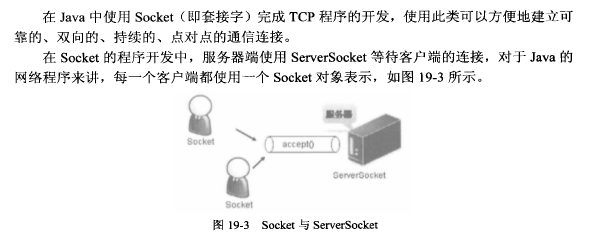
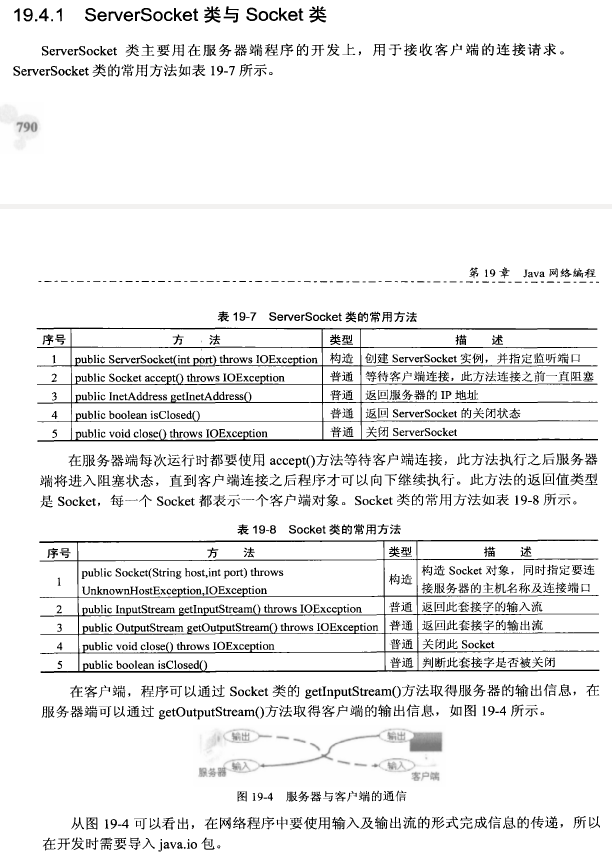
1 | import java.io.PrintStream; |
1 | import java.io.BufferedReader; |
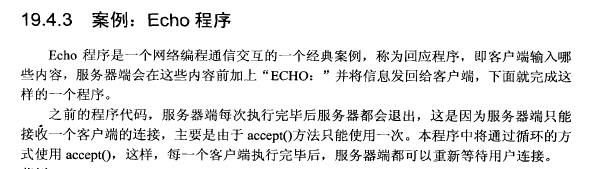
1 | import java.io.BufferedReader; |
1 | import java.io.BufferedReader; |

1 | import java.io.BufferedReader; |
4.UDP#
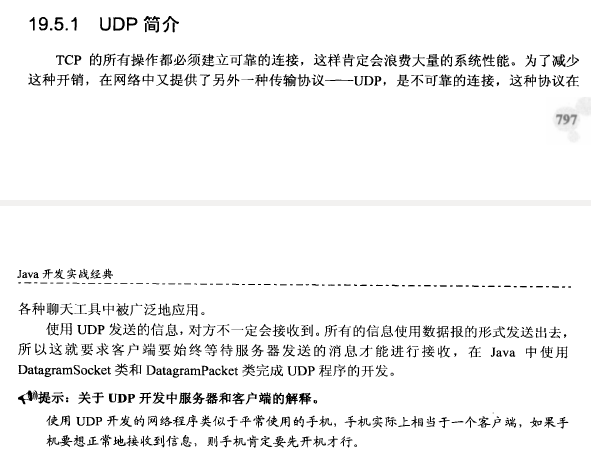
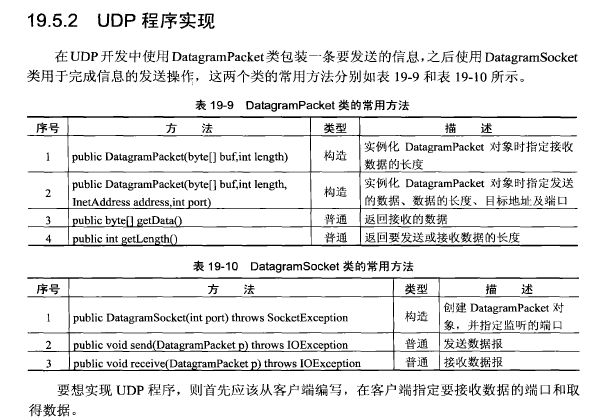
UDP server
1 | import java.net.DatagramPacket; |
UDP client
1 | import java.net.DatagramPacket; |