1.请安装好Go的环境,参考上一篇open-falcon的安装博文
2.安装** mail-provider**
1 | https://github.com/open-falcon/mail-provider |
安装方法
1 | cd $GOPATH/src |
编译成功之后,修改cfg.json文件相关信息,使用
1 | ./control start |
在cfg.json里面使用的163邮箱的smtp服务,需要开启客户端授权码,如果提示:发送数量超过配额,请过24小时再请求手机验证码
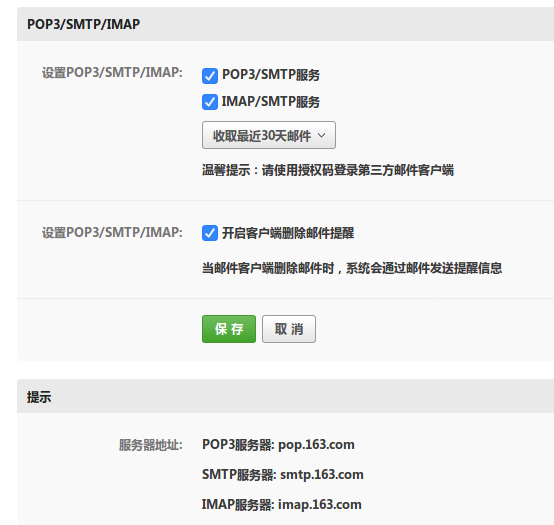
1.请安装好Go的环境,参考上一篇open-falcon的安装博文
2.安装** mail-provider**
1 | https://github.com/open-falcon/mail-provider |
安装方法
1 | cd $GOPATH/src |
编译成功之后,修改cfg.json文件相关信息,使用
1 | ./control start |
在cfg.json里面使用的163邮箱的smtp服务,需要开启客户端授权码,如果提示:发送数量超过配额,请过24小时再请求手机验证码
在Ubuntu下安装open-falcon和Centos下安装的方法有点区别,因为Ubuntu使用的包管理器是apt-get,而Centos下使用的是Yum,建议不要再Ubuntu下使用yum
建议自己下载源码打包二进制包来安装,因为官方给出的二进制包应该是再centos下打包的,再Ubuntu下运行可能会出现问题
1.安装Go,首先去官网下载,需要fq
1 | https://golang.org |
顺便安装Goland,注册服务器 http://idea.youbbs.org
2.在/etc/profile中添加,后source /etc/profile
1 | #Go |
验证是否安装成功
1 | go version |
接下来参考
1.首先你需要知道flume的http监控端口是否启动
请参考博文 Flume的监控参数
即在 http://localhost:3000/metrics 可以访问到如下内容
2.在open-falcon中安装flume监控插件,参考官方文档 http://book.open-falcon.org/zh_0_2/usage/flume.html
官方文档写的很不清楚,请参考本文接下来给出的步骤
首先修改agent的配置文件,agent负责的是采集数据,同时有调度脚本插件的功能
1 | ~/software/open-falcon-v0.2.1/agent/config |
修改如下,即写入了flume监控脚本的git地址,在此感谢插件作者在学习过程中的指导
1.首先需要注册一个网易的邮箱,开启smtp服务,并使用其授权码
2.发送邮件的Python脚本
1 | #!/usr/bin/python |
如果想美化邮件,可以在其中添加html样式,并将邮件的格式指定成html
1 | content = """ |
邮件样式如下
普通的flume启动命令
1 | bin/flume-ng agent -c conf -f conf/flume-conf.properties -n agent -Dflume.root.logger=INFO,console |
日志信息在终端输出,只有去掉这个参数,日志才能在log4j和logback中输出
1 | -Dflume.root.logger=INFO,console |
如果要加上http监控的话
1 | bin/flume-ng agent -c conf -f conf/flume-conf.properties -n agent -Dflume.root.logger=INFO,console -Dflume.monitoring.type=http -Dflume.monitoring.port=34545 |
即加上参数,flume.monitoring.type=http 指定了Reporting的方式为http,flume.monitoring.port 指定了http服务的端口号
1 | -Dflume.monitoring.type=http -Dflume.monitoring.port=34545 |
参考 : kafka管理器kafka-manager部署安装
下载Kafka Manager,并进行打包,由于Kafka manager是由scala写的,所以需要由sbt的支持
1 | git clone https://github.com/yahoo/kafka-manager |
关于sbt的的安装,请移步
1 | http://www.cnblogs.com/tonglin0325/p/8884470.html |
配置zk的地址
1 | 在conf/application.conf中将kafka-manager.zkhosts的值设置为localhost |
打包完成后,对kafka-manager-1.3.3.17.zip包进行解压,位置在
1 | kafka-manager/target/universal |
参考: http://www.cnblogs.com/EasonJim/p/7130171.html
安装indicator-sysmonitor
1 | sudo add-apt-repository ppa:fossfreedom/indicator-sysmonitor |
或者直接下载deb包
1 | https://launchpad.net/indicator-sysmonitor/+download |
启动:
indicator-sysmonitor &
添加
cpu: {cpu} 内存: {mem} 网络: {net}保存
1 | https://amazonmsk.s3.amazonaws.com/MSK_Sizing_Pricing.xlsx |
1、下载sbt通用平台压缩包:sbt-0.13.5.tgz
1 | http://www.scala-sbt.org/download.html |
2、建立目录,解压文件到所建立目录
1 | $ sudo tar zxvf sbt-0.13.5.tgz -C /opt/scala/ |
3、建立启动sbt的脚本文件
1 | /*选定一个位置,建立启动sbt的脚本文本文件,如/opt/scala/sbt/ 目录下面新建文件名为sbt的文本文件*/ |
参考 ubuntu16.04 配置shadowsocks及使用教程,支持chacha20-ietf-poly1305加密方式
1 | sudo apt-get install software-properties-common -y |
1 | sudo vi /etc/shadowsocks-libev.json |
内容
1 | { |
1 | ss-local -c /etc/shadowsocks-libev.json & |
Centos下安装
参考
1 | https://gist.github.com/aa65535/ea090063496b0d3a1748 |