1.查询PrestoDB(facebook版本)#
1.创建PrestoDB环境#
使用docker创建presto测试环境
1 | https://hub.docker.com/r/prestodb/presto/tags |
拉取镜像
1 | docker pull prestodb/presto:0.284 |
启动
1 | docker run -p 8080:8080 -ti -v /Users/lintong/Downloads/config.properties:/opt/presto-server/etc/config.properties -v /Users/lintong/Downloads/jvm.config:/opt/presto-server/etc/jvm.config prestodb/presto:0.284 |
其中config.properties配置文件
1 | coordinator=true |
jvm.config
1 | -server |
配置参考:https://github.com/prestodb/presto/tree/master/docker/etc
https://prestodb.github.io/docs/current/installation/deployment.html
访问localhost:8080
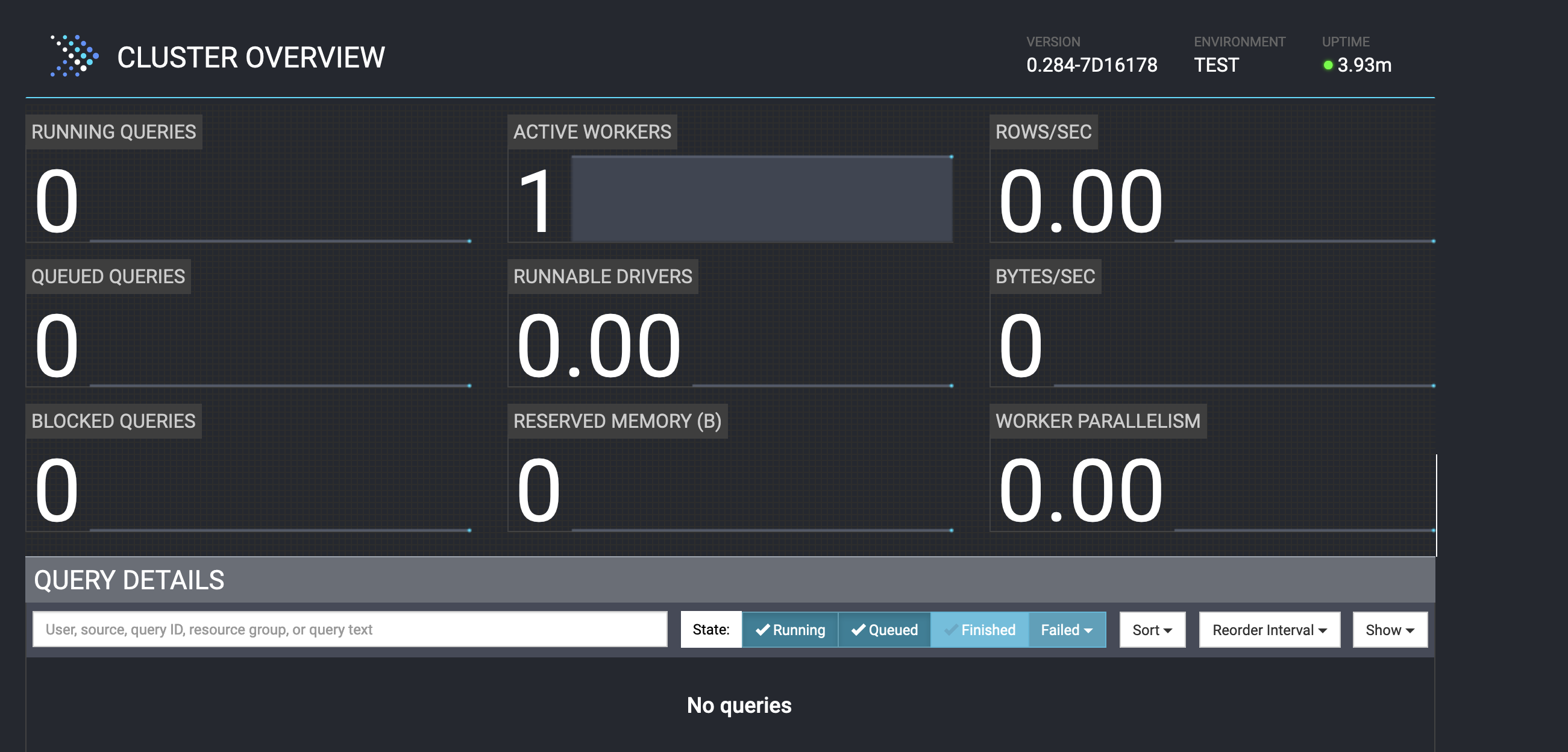
2.使用client连接PrestoDB#
可以使用presto-go-client,官方文档
1 | https://github.com/prestodb/presto-go-client |
引入依赖
1 | go get github.com/prestodb/presto-go-client/presto |
go连接数据库使用的是database/sql,还需要额外引入具体数据库的driver,比如
presto的github.com/prestodb/presto-go-client/presto
mysql的github.com/go-sql-driver/mysql
impala的github.com/bippio/go-impala
查询presto代码
1 | import ( |
参考:golang+presto查询在数据平台中ad hoc查询
如果想同时查询多行的话,也可以先定义一个struct
1 | type YourTable struct { |
然后在迭代查询的时候使用这个struct
1 | // 迭代结果行 |
3.使用REST API连接PrestoDB#
参考官方文档
1 | https://prestodb.io/docs/current/develop/client-protocol.html |
其实presto-go-client底层也是使用presto的REST API的POST请求来提交任务,参考源码
1 | https://github.com/prestodb/presto-go-client/tree/master/presto#L635 |
2.查询Trino(社区版本)#
1.创建Trino环境#
创建Trino环境用于测试可以使用docker
1 | https://hub.docker.com/r/trinodb/trino/tags |
拉取镜像
1 | docker pull trinodb/trino:435 |
启动
1 | docker run -p 8080:8080 -ti trinodb/trino:435 |
访问localhost:8080,默认密码不启用,随便输入一个用户名
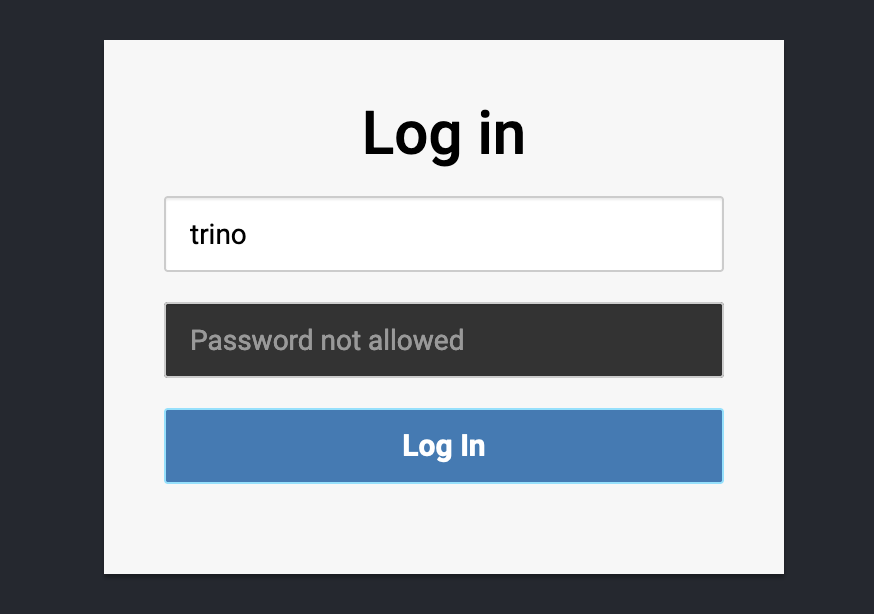
界面
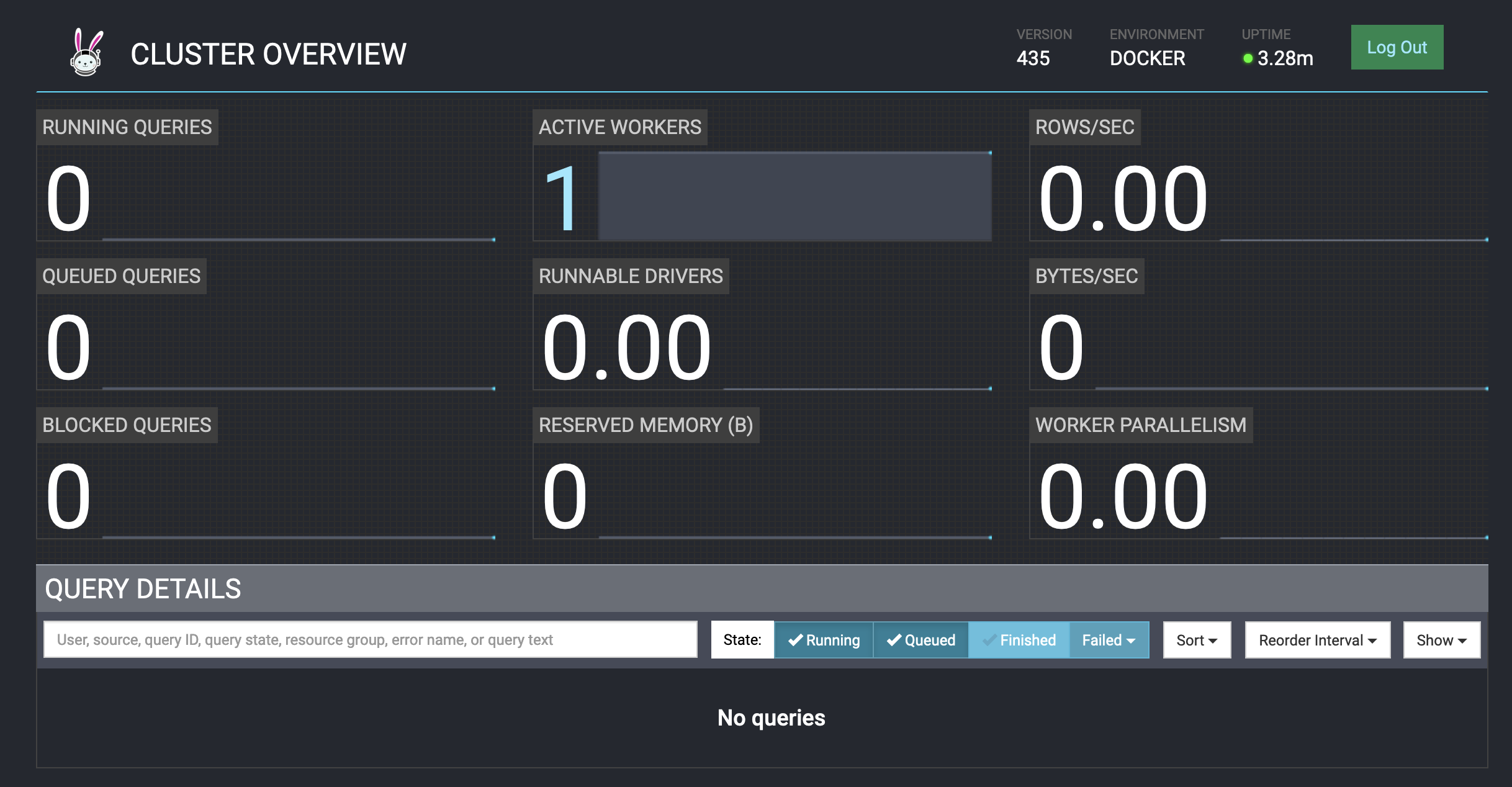
2.使用client连接trino#
可以使用trino-go-client,官方文档
1 | https://github.com/trinodb/trino-go-client |
引入依赖
1 | go get github.com/trinodb/trino-go-client/trino |
查询trino代码
1 | import ( |
3.使用REST API连接TrinoDB#
参考官方文档
1 | https://trino.io/docs/current/develop/client-protocol.html |
其实trino-go-client底层也是使用trino的REST API的POST请求来提交任务,参考源码
1 | https://github.com/trinodb/trino-go-client/blob/master/trino/trino.go#L820 |